Flood Fill
Problem Statement
An image is represented by an m x n
integer grid image
where image[i][j]
represents the pixel value of the image.
You are also given three integers sr
, sc
, and color
. You should perform a flood fill on the image starting from the pixel image[sr][sc]
.
To perform a flood fill, consider the starting pixel, plus any pixels connected 4-directionally to the starting pixel of the same color as the starting pixel, plus any pixels connected 4-directionally to those pixels (also with the same color), and so on. Replace the color of all of the aforementioned pixels with color
.
Return the modified image after performing the flood fill.
You can submit the problem here.
Example 1
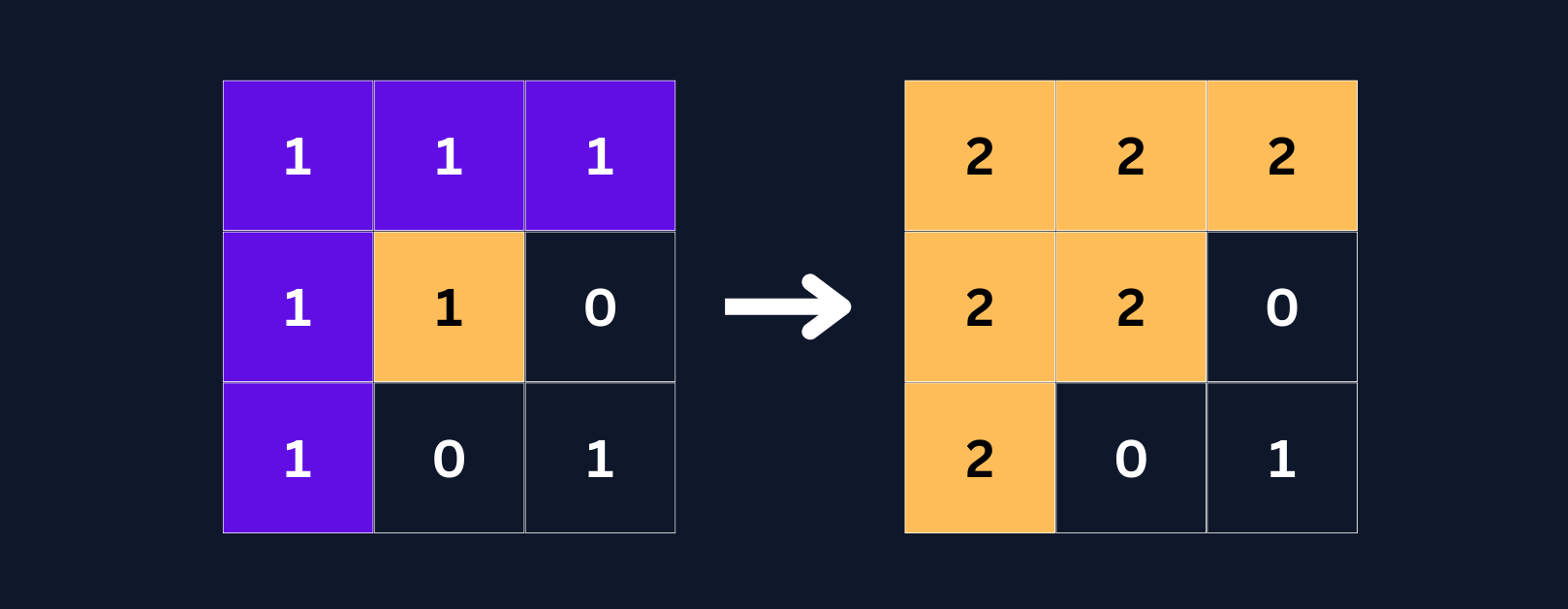
Input: image = [[1,1,1],[1,1,0],[1,0,1]], sr = 1, sc = 1, color = 2
Output: [[2,2,2],[2,2,0],[2,0,1]]
Explanation: From the center of the image with position (sr, sc) = (1, 1) (i.e., the red pixel), all pixels connected by a path of the same color as the starting pixel (i.e., the blue pixels) are colored with the new color.
Note the bottom corner is not colored 2, because it is not 4-directionally connected to the starting pixel.
Example 2
Input: image = [[0,0,0],[0,0,0]], sr = 0, sc = 0, color = 0
Output: [[0,0,0],[0,0,0]]
Explanation: The starting pixel is already colored 0, so no changes are made to the image.
Java Code
class Solution {
private int[][] directions = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}};
public void dfs(int row, int col, int[][] image, int oldColor, int color) {
if (row < 0 || row >= image.length || col < 0 || col >= image[0].length || image[row][col] != oldColor) {
return;
}
image[row][col] = color;
for (int[] direction : directions) {
int newRow = row + direction[0];
int newCol = col + direction[1];
dfs(newRow, newCol, image, oldColor, color);
}
}
public int[][] floodFill(int[][] image, int sr, int sc, int color) {
if (image[sr][sc] == color) return image;
dfs(sr, sc, image, image[sr][sc], color);
return image;
}
}
C++ Code
class Solution {
public:
vector<vector<int>> directions = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}};
void dfs(int row, int col, vector<vector<int>>& image, int oldColor, int color) {
if(row < 0 || row >= image.size() || col < 0 || col >= image[0].size() || image[row][col] != oldColor) {
return;
}
image[row][col] = color;
for(auto direction : directions) {
int new_row = row + direction[0];
int new_col = col + direction[1];
dfs(new_row, new_col, image, oldColor, color);
}
}
vector<vector<int>> floodFill(vector<vector<int>>& image, int sr, int sc, int color) {
if(image[sr][sc] == color) return image;
dfs(sr, sc, image, image[sr][sc], color);
return image;
}
};
Python Code
class Solution:
def __init__(self):
self.directions = [[-1, 0], [1, 0], [0, -1], [0, 1]]
def dfs(self, row, col, image, oldColor, color):
if (row < 0 or row >= len(image) or col < 0 or col >= len(image[0]) or image[row][col] != oldColor):
return
image[row][col] = color
for direction in self.directions:
new_row = row + direction[0]
new_col = col + direction[1]
self.dfs(new_row, new_col, image, oldColor, color)
def floodFill(self, image: List[List[int]], sr: int, sc: int, color: int) -> List[List[int]]:
if image[sr][sc] == color:
return image
self.dfs(sr, sc, image, image[sr][sc], color)
return image
Javascript Code
var floodFill = function(image, sr, sc, color) {
const directions = [[-1, 0], [1, 0], [0, -1], [0, 1]];
const dfs = (row, col, oldColor) => {
if (row < 0 || row >= image.length || col < 0 || col >= image[0].length || image[row][col] !== oldColor) {
return;
}
image[row][col] = color;
for (const direction of directions) {
const newRow = row + direction[0];
const newCol = col + direction[1];
dfs(newRow, newCol, oldColor);
}
};
if (image[sr][sc] === color) return image;
dfs(sr, sc, image[sr][sc]);
return image;
};
Go Code
func floodFill(image [][]int, sr, sc, color int) [][]int {
directions := [][]int{{-1, 0}, {1, 0}, {0, -1}, {0, 1}}
var dfs func(row, col, oldColor int)
dfs = func(row, col, oldColor int) {
if row < 0 || row >= len(image) || col < 0 || col >= len(image[0]) || image[row][col] != oldColor {
return
}
image[row][col] = color
for _, direction := range directions {
newRow := row + direction[0]
newCol := col + direction[1]
dfs(newRow, newCol, oldColor)
}
}
if image[sr][sc] == color {
return image
}
dfs(sr, sc, image[sr][sc])
return image
}
Complexity Analysis
- Time Complexity:
O(N)
, whereN
is the number of pixels in the image. We might process every pixel. - Space Complexity:
O(N)
, the size of the implicit call stack when callingdfs()
.
You may follow us on Social Media.