Problem Statement
Given n
non-negative integers representing an elevation map where the width of each bar is 1
, compute how much water it can trap after raining.
Example 1
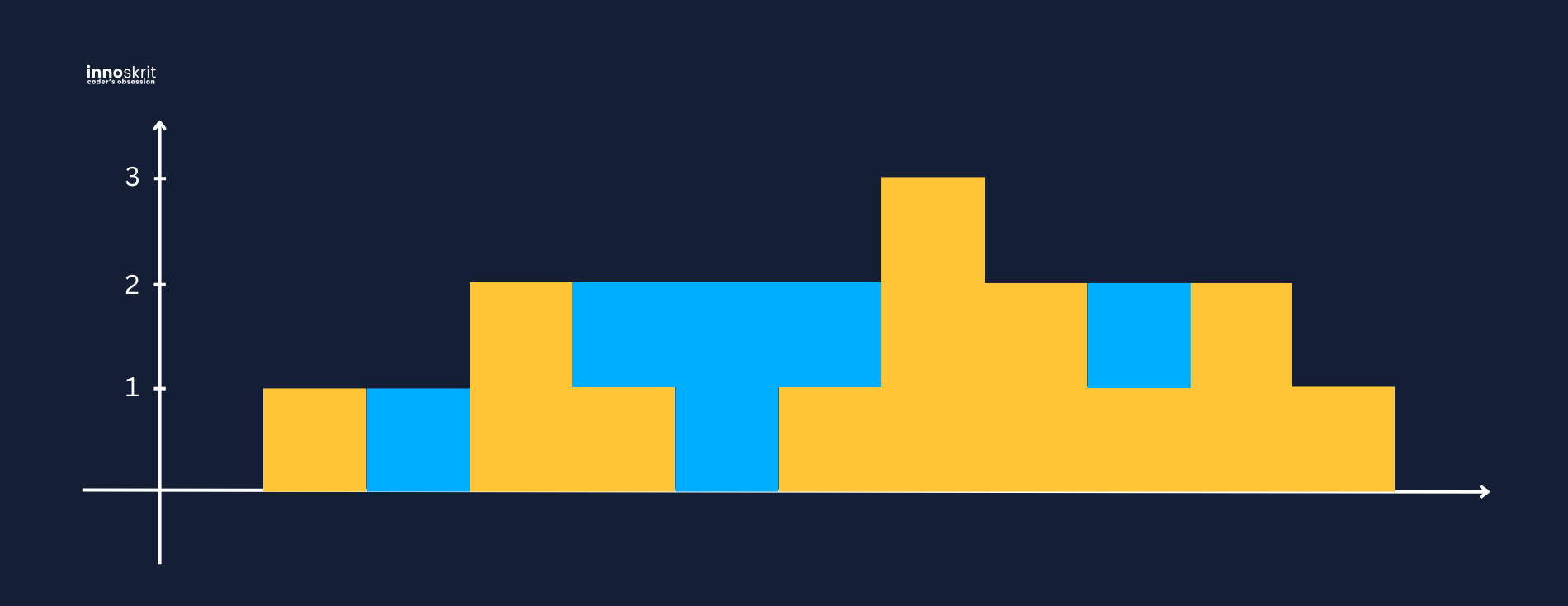
Input: height = [0,1,0,2,1,0,1,3,2,1,2,1]
Output: 6
Explanation: The above elevation map (black section) is represented by array [0,1,0,2,1,0,1,3,2,1,2,1]. In this case, 6 units of rain water (blue section) are being trapped.
Example 2
Input: height = [4,2,0,3,2,5]
Output: 9
Try here before watching the video.
Video Solution
Java Code
class Solution {
public int trap(int[] height) {
int left = 0, right = height.length - 1, leftMax = 0, rightMax = 0;
int ans = 0;
while(left < right) {
if(height[left] <= height[right]) {
if(leftMax < height[left]) {
leftMax = height[left];
} else {
ans += (leftMax - height[left]);
}
left += 1;
} else {
if(rightMax <=height[right]) {
rightMax = height[right];
} else {
ans += (rightMax - height[right]);
}
right -= 1;
}
}
return ans;
}
}
C++ Code
class Solution {
public:
int trap(vector<int>& height) {
int left = 0, right = height.size() - 1, leftMax = 0, rightMax = 0;
int ans = 0;
while(left < right) {
if(height[left] <= height[right]) {
if(leftMax < height[left]) {
leftMax = height[left];
} else {
ans += (leftMax - height[left]);
}
left += 1;
} else {
if(rightMax <= height[right]) {
rightMax = height[right];
} else {
ans += (rightMax - height[right]);
}
right -= 1;
}
}
return ans;
}
};
Python Code
class Solution:
def trap(self, height: List[int]) -> int:
left, right = 0, len(height) - 1
leftMax, rightMax = 0, 0
ans = 0
while left < right:
if height[left] <= height[right]:
if leftMax < height[left]:
leftMax = height[left]
else:
ans += leftMax - height[left]
left += 1
else:
if rightMax <= height[right]:
rightMax = height[right]
else:
ans += rightMax - height[right]
right -= 1
return ans
Javascript Code
var trap = function(height) {
let left = 0, right = height.length - 1;
let leftMax = 0, rightMax = 0;
let ans = 0;
while (left < right) {
if (height[left] <= height[right]) {
if (leftMax < height[left]) {
leftMax = height[left];
} else {
ans += leftMax - height[left];
}
left++;
} else {
if (rightMax <= height[right]) {
rightMax = height[right];
} else {
ans += rightMax - height[right];
}
right--;
}
}
return ans;
};
Go Code
func trap(height []int) int {
left, right := 0, len(height)-1
leftMax, rightMax := 0, 0
ans := 0
for left < right {
if height[left] <= height[right] {
if leftMax < height[left] {
leftMax = height[left]
} else {
ans += leftMax - height[left]
}
left++
} else {
if rightMax <= height[right] {
rightMax = height[right]
} else {
ans += rightMax - height[right]
}
right--
}
}
return ans
}
Complexity Analysis
Time Complexity: O(N)
Space Complexity: O(1)