Problem Statement
Given the head
of a singly linked list, reverse the list, and return the reversed list.
Example
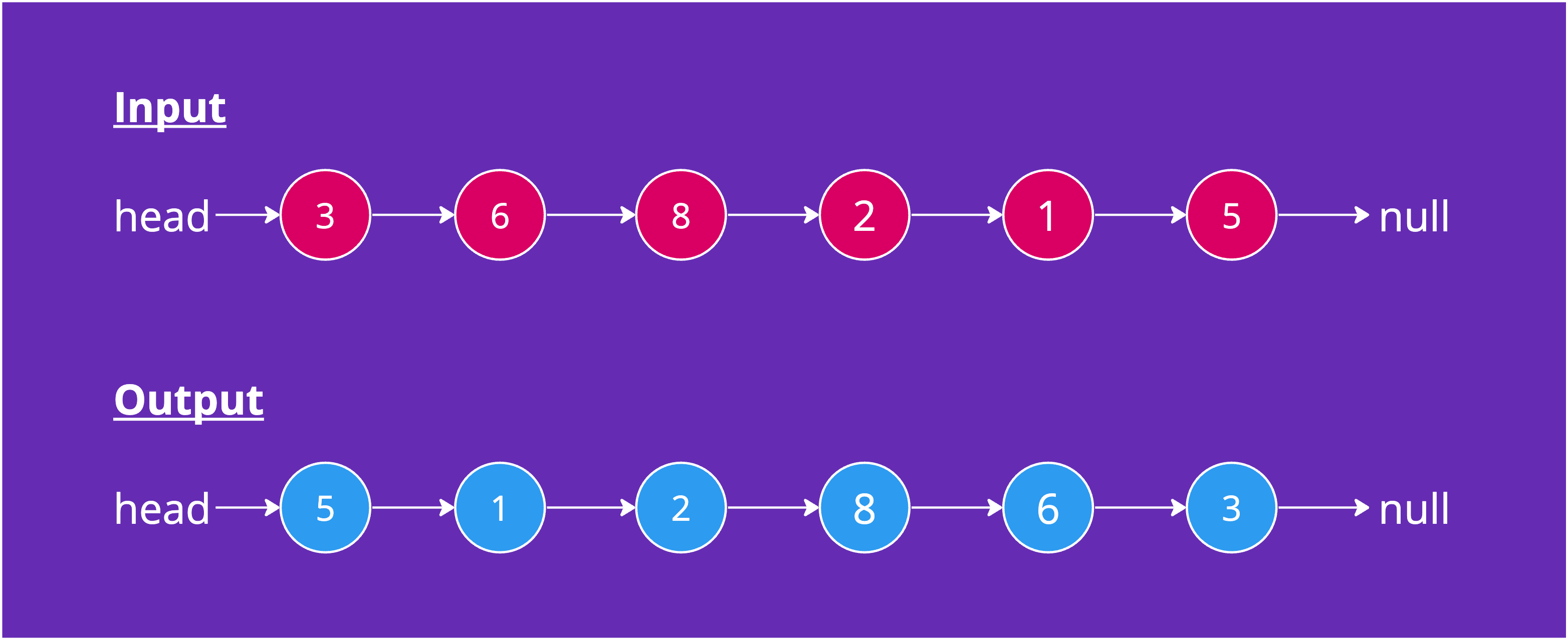
Input: head = [3,6,8,2,1,5]
Output: [5,1,2,8,6,3]
Try here before watching the video.
Video Explanation
Java Code
class Solution {
public ListNode reverseList(ListNode head) {
ListNode prev, curr, next;
prev = next = null;
curr = head;
while(curr != null) {
next = curr.next;
curr.next = prev;
prev = curr;
curr = next;
}
head = prev;
return head;
}
}
C++ Code
class Solution {
public:
ListNode* reverseList(ListNode* head) {
ListNode* prev = nullptr;
ListNode* curr = head;
ListNode* next = nullptr;
while (curr != nullptr) {
next = curr->next;
curr->next = prev;
prev = curr;
curr = next;
}
head = prev;
return head;
}
};
Python Code
class Solution:
def reverseList(self, head: Optional[ListNode]) -> Optional[ListNode]:
prev = None
curr = head
next = None
while curr:
next = curr.next
curr.next = prev
prev = curr
curr = next
head = prev
return head
Javascript Code
var reverseList = function(head) {
let prev = null;
let curr = head;
let next = null;
while (curr !== null) {
next = curr.next;
curr.next = prev;
prev = curr;
curr = next;
}
head = prev;
return head;
};
Go Code
func reverseList(head *ListNode) *ListNode {
var prev *ListNode
curr := head
var next *ListNode
for curr != nil {
next = curr.Next
curr.Next = prev
prev = curr
curr = next
}
head = prev
return head
}
Complexity Analysis
Time Complexity: O(N), we are iterating over the complete linked list
Space Complexity: O(1), we are not taking any extra space.