Java
Print Sum in Range using Two Threads in Java
Printing sum in range using two threads is one of the very important questions of software interviews.
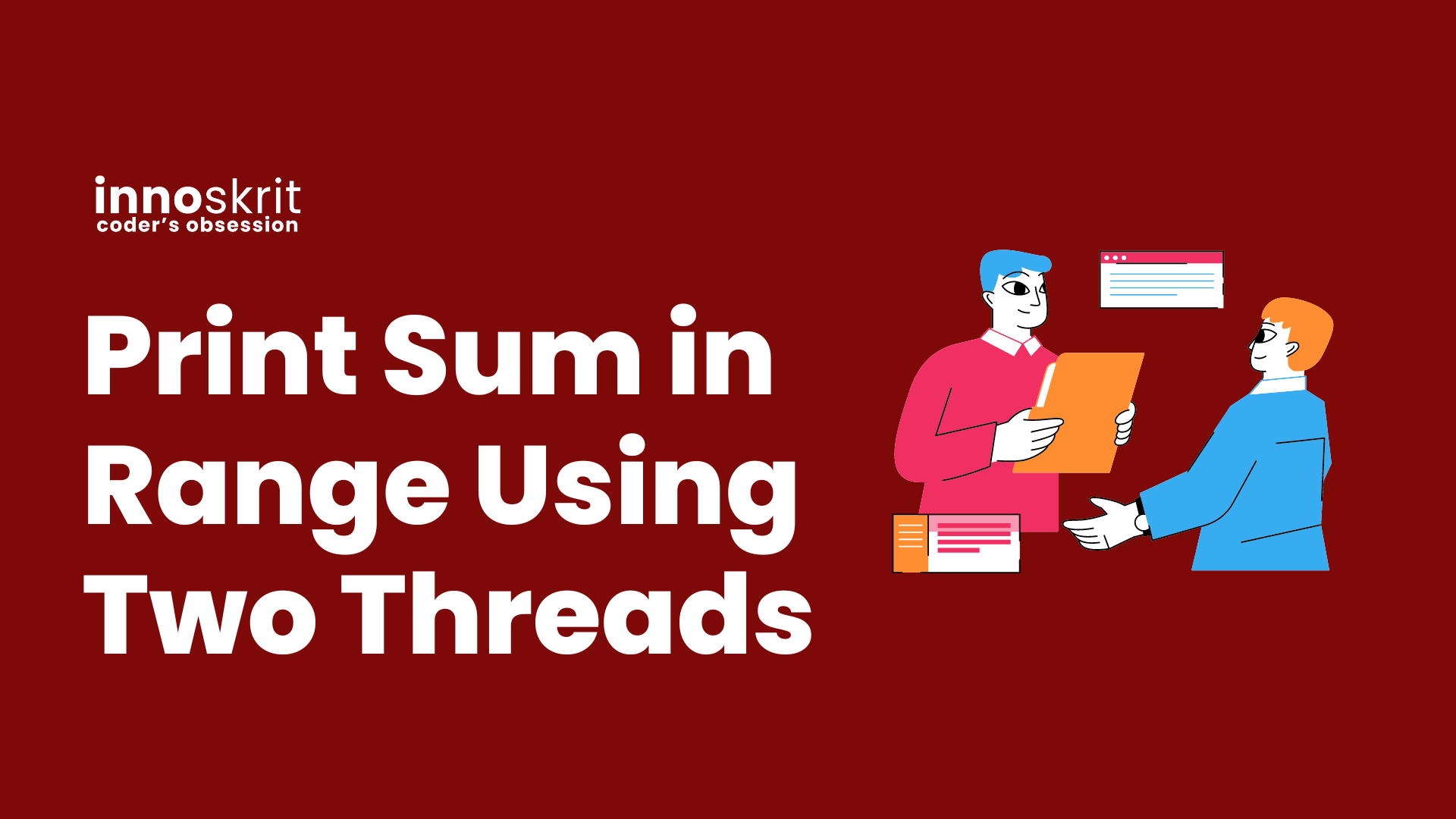
Video Explanation
Video Explanation
Let’s first try to find the sum in range using main thread only.
Java program which calculates the sum in range using main thread.
class Adder {
int start;
int end;
int counter;
public Adder(int start, int end) {
this.start = start;
this.end = end;
this.counter = 0;
}
public void add() {
for(int i = this.start; i <= this.end; i++) {
this.counter += i;
}
}
public static void main(String[] args) {
Adder adder = new Adder(1, 10000);
adder.add();
System.out.println(adder.counter);
}
}
Output
50005000
Let’s now try to find the sum in range using two threads.
Java program which calculates the sum in range using two threads.
public class ConcurrentAdder {
int start;
int end;
int counter;
public ConcurrentAdder(int start, int end) {
this.start = start;
this.end = end;
this.counter = 0;
}
public void add() {
for(int i = this.start; i <= this.end; i++) {
this.counter += i;
}
}
public static void main(String[] args) throws InterruptedException {
ConcurrentAdder adder1 = new ConcurrentAdder(1, 5000);
ConcurrentAdder adder2 = new ConcurrentAdder(5001, 10000);
Thread t1 = new Thread(() -> {
adder1.add();
});
Thread t2 = new Thread(() -> {
adder2.add();
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println(adder1.counter + adder2.counter);
}
}
Output
50005000
We hope you have learnt something new.